String is Immutable - String Vs String builder
String is immutable
- String a=”a”; address is 12345
- a=”b”; address will be 3456
- String a=new String(“a”);
- Will create one object in String pool and create one more object for new operator will get created and that will point to it.
- String a=”a”; will create object in string pool and object a will point to it.
StringBuffer is mutable
- StringBuffer b=”a”; address is 12345
- b=”b”; address is 12345
- This is synchronized
StringBuilder : This is similar to StringBuffer but this is not synchronized
Inner join Vs Outer join
This is most common interview question for many developers ... I would like to give a easy understanding of this to remember for life ...
- An inner join of A and B gives the result of A intersect B, i.e. the inner part of a venn diagram intersection.
- An outer join of A and B gives the results of A union B, i.e. the outer parts of a venn diagram union.
Suppose you have two Tables, with a single column each, and data as follows:
A B
- -
1 3
2 4
3 5
4 6
Note that (1,2) are unique to A, (3,4) are common, and (5,6) are unique to B.
Inner join
An inner join using either of the equivalent queries gives the intersection of the two tables, i.e. the two rows they have in common.
select * from a INNER JOIN b on a.a = b.b;
select a.*,b.* from a,b where a.a = b.b;
a | b
--+--
3 | 3
4 | 4
Left outer join
A left outer join will give all rows in A, plus any common rows in B.
select * from a LEFT OUTER JOIN b on a.a = b.b;
select a.*,b.* from a,b where a.a = b.b(+);
a | b
--+-----
1 | null
2 | null
3 | 3
4 | 4
Full outer join
A full outer join will give you the union of A and B, i.e. All the rows in A and all the rows in B. If something in A doesn't have a corresponding datum in B, then the B portion is null, and vice versa.
select * from a FULL OUTER JOIN b on a.a = b.b;
a | b
-----+-----
1 | null
2 | null
3 | 3
4 | 4
null | 6
null | 5
Inner join
Full Outer join
Reference : [1]
The dictionary definition of polymorphism refers to a principle in biology in which an organism or species can have many different forms or stages. This principle can also be applied to object-oriented programming and languages like the Java language. Subclasses of a class can define their own unique behaviors and yet share some of the same functionality of the parent class.
Polymorphism can exists in two ways... 1. Static polymorphism - Overloading
2. Dynamic polymorphism - Overriding
Overloading : Creating mutiple methods with same name in a class but with different signatures (either number of parameters should be different or type of parameters should be different in each method)
Overriding : Creating same method same parameters but with different behavior in both super class and extended class is called as overriding.
Dynamic binding is nothing but choosing which method to chose when super class object is created with extended class type.
Please have a look at the below program and output to understand the polymorphisms...
public class Ploymorphism {
public interface Vegetarian{
void eatVeg();
}
public class Animal{
void eat(){
System.out.println("All animals can eat");
}
}
public class Deer extends Animal implements Vegetarian{
public void eat(String veg) {
System.out.println("I am eating :"+veg);
}
public void eatVeg(){
System.out.println("I eat only veg");
}
}
public class Tiger extends Animal{
void eat(){
System.out.println("I'm tiger, I eat what I want");
}
void eat(String veg, String nonveg){
System.out.println("I can eat:"+veg+" and "+nonveg);
}
}
public static void main(String[] args) {
System.out.println("Java has two types of polymorphism");
System.out.println("1.Static Polymorphism : Achived using \"overloading\" ");
Ploymorphism p = new Ploymorphism();
Tiger tiger = p.new Tiger();
tiger.eat("leaves", "creatures");
System.out.println("Tiger has two methods with same name but with different paramaetrs/ signatiures(either no of parameters should be different or type of paramters should be different)");
System.out.println("2.Dynamic Polymorphism : Achived using \"overriding\" ");
Deer deer = p.new Deer();
deer.eat("carrot");
System.out.println("deer is extended from animal but eat method has different in the deer than animal class- it is overriding");
Animal whiteTiger = p.new Tiger();
whiteTiger.eat();
//whiteTiger.eat("prawns","fish");
//System.out.println("The above commented line gives error as there is no eat method of such type in the animal class");
}
}
Ouput of the above program :
Java has two types of polymorphism
1.Static Polymorphism : Achived using "overloading"
I can eat:leaves and creatures
Tiger has two methods with same name but with different paramaetrs/ signatiures(either no of parameters should be different or type of paramters should be different)
2.Dynamic Polymorphism : Achived using "overriding"
I am eating :carrot
deer is extended from animal but eat method has different in the deer than animal class- it is overriding
I'm tiger, I eat what I want
Interfaces: These are abstract data types that represent collections. Interfaces allow collections to be manipulated independently of the details of their representation. In object-oriented languages, interfaces generally form a hierarchy.
Implementations: These are the concrete implementations of the collection interfaces. In essence, they are reusable data structures.
Algorithms: These are the methods that perform useful computations, such as searching and sorting, on objects that implement collection interfaces. The algorithms are said to be polymorphic: that is, the same method can be used on many different implementations of the appropriate collection interface. In essence, algorithms are reusable functionality.
- Reduces programming effort: By providing useful data structures and algorithms, the Collections Framework frees you to concentrate on the important parts of your program rather than on the low-level "plumbing" required to make it work. By facilitating interoperability among unrelated APIs, the Java Collections Framework frees you from writing adapter objects or conversion code to connect APIs.
- Increases program speed and quality: This Collections Framework provides high-performance, high-quality implementations of useful data structures and algorithms. The various implementations of each interface are interchangeable, so programs can be easily tuned by switching collection implementations. Because you're freed from the drudgery of writing your own data structures, you'll have more time to devote to improving programs' quality and performance.
- Allows interoperability among unrelated APIs: The collection interfaces are the vernacular by which APIs pass collections back and forth. If my network administration API furnishes a collection of node names and if your GUI toolkit expects a collection of column headings, our APIs will interoperate seamlessly, even though they were written independently.
- Reduces effort to learn and to use new APIs: Many APIs naturally take collections on input and furnish them as output. In the past, each such API had a small sub-API devoted to manipulating its collections. There was little consistency among these ad hoc collections sub-APIs, so you had to learn each one from scratch, and it was easy to make mistakes when using them. With the advent of standard collection interfaces, the problem went away.
- Reduces effort to design new APIs: This is the flip side of the previous advantage. Designers and implementers don't have to reinvent the wheel each time they create an API that relies on collections; instead, they can use standard collection interfaces.
- Fosters software reuse: New data structures that conform to the standard collection interfaces are by nature reusable. The same goes for new algorithms that operate on objects that implement these interfaces.
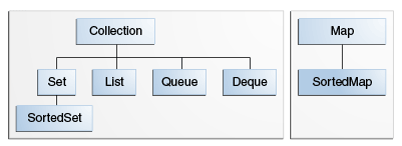
- The Collection interface provides support for the implementation of a mathematical bag - an unordered collection of objects that may contain duplicates.
- HashTable : Whenever a program wants to store a key value pair, one can use Hashtable.
- Set : The Set interface provides methods for accessing the elements of a finite mathematical set. Sets do not allow duplicate elements.
- Implements Collection Interface
- Does not allow duplicate
- EnumSet (classes)
- HashSet (classes) : HashSet uses map to store. It stores element as KEY and "PRESENT" object as VALUE.
- Sortedset (Interface)
- Ascending order
- Does not allow duplicate
- TreeSet (classes)
- LinkedHashSet (classes)
- List : The List interface provides support for ordered collections of objects.
- Implement collection Interface
- Can contain duplicate and it’s ordered
- Stack class implements list interface
- Linked List class implements list interface
- Vector (classes) - Syncronized : The Vector class provides the capability to implement a growable array of objects.Yes a Vector can contain heterogenous objects. Because a Vector stores everything in terms of Object.
- ArrayList (classes) - nonsynchronized . Yes a ArrayList can contain heterogenous objects. Because a ArrayList stores everything in terms of Object.
- The basic difference between a Vector and an ArrayList is that, vector is synchronized while ArrayList is not. Thus whenever there is a possibility of multiple threads accessing the same instance, one should use Vector. While if not multiple threads are going to access the same instance then use ArrayList. Non synchronized data structure will give better performance than the synchronized one.
- How to sort list in reverse order?How to sort list in reverse order?
- This question is just like above to test your knowledge of Collections utility class. Use it reverse() method to reverse the list.
List reversedList = Collections.reverse(list); - cc
- Queue
- De-queue:
- Map: Map interface is a special type of collection which is used to store key-value pairs. It does not extend Collection interface for this reason.Main classes implementing Map interface are:
- HashMap,
- Hashtable,
- EnumMap,
- IdentityHashMap,
- LinkedHashMap,
- Properties.
- When to use HashMap or TreeMap?
- TreeMap is special form of HashMap. It maintains the ordering of keys which is missing in HashMap class. This ordering is by default “natural ordering”. The default ordering can be override by providing an instance of Comparator class, whose compare method will be used to maintain ordering of keys.
- SortedMap :

- To remember :
- Legacy collection frame works are Synchronized:
- Vector
- stack
- dictionary
- hashTable
- Properties
- New collection framework are not synchronized:
- LinkedList
- ArrayList
- HashSet
- TreeSet
- HashMap
- TreeMap
Difference between Set and List?
The most noticeable differences are :- Set is unordered collection where List is ordered collection based on zero based index.
- List allow duplicate elements but Set does not allow duplicates.
- List does not prevent inserting null elements (as many you like), but Set will allow only one null element.
Perhaps most easy question. List is collection of elements where as map is collection of key-value pairs. There is actually lots of differences which originate from first statement. They have separate top level interface, separate set of generic methods, different supported methods and different views of collection.
I will take much time hear as answer to this question is enough as first difference only.
Difference between HashMap and HashTable?
There are several differences between HashMap and Hashtable in Java:
- Hashtable is synchronized, whereas HashMap is not.
- Hashtable does not allow null keys or values. HashMap allows one null key and any number of null values.
- The third significant difference between HashMap vs Hashtable is that Iterator in the HashMap is a fail-fast iterator while the enumerator for the Hashtable is not.
Lets note down the differences:
- All the methods of Vector is synchronized. But, the methods of ArrayList is not synchronized.
- Vector is a Legacy class added in first release of JDK. ArrayList was part of JDK 1.2, when collection framework was introduced in java.
- By default, Vector doubles the size of its array when it is re-sized internally. But, ArrayList increases by half of its size when it is re-sized.
Iterators differ from enumerations in three ways:
- Iterators allow the caller to remove elements from the underlying collection during the iteration with its remove() method. You can not add/remove elements from a collection when using enumerator.
- Enumeration is available in legacy classes i.e Vector/Stack etc. whereas Iterator is available in all modern collection classes.
- Another minor difference is that Iterator has improved method names e.g. Enumeration.hasMoreElement() has become Iterator.hasNext(), Enumeration.nextElement() has become Iterator.next() etc.
HashMap is collection of key-value pairs whereas HashSet is un-ordered collection of unique elements. That’s it. No need to describe further.
Difference between Iterator and ListIterator?
There are three Differences are there:
- We can use Iterator to traverse Set and List and also Map type of Objects. But List Iterator can be used to traverse for List type Objects, but not for Set type of Objects.
- By using Iterator we can retrieve the elements from Collection Object in forward direction only whereas List Iterator, which allows you to traverse in either directions using hasPrevious() and previous() methods.
- ListIterator allows you modify the list using add() remove() methods. Using Iterator you can not add, only remove the elements.
SortedSet is an interface which TreeSet implements. That’ it !!
Difference between ArrayList and LinkedList?
- LinkedList store elements within a doubly-linked list data structure. ArrayList store elements within a dynamically resizing array.
- LinkedList allows for constant-time insertions or removals, but only sequential access of elements. In other words, you can walk the list forwards or backwards, but grabbing an element in the middle takes time proportional to the size of the list. ArrayLists, on the other hand, allow random access, so you can grab any element in constant time. But adding or removing from anywhere but the end requires shifting all the latter elements over, either to make an opening or fill the gap.
- LinkedList has more memory overhead than ArrayList because in ArrayList each index only holds actual object (data) but in case of LinkedList each node holds both data and address of next and previous node.
To create a mutable class in Java you have to make sure the following requirements are satisfied:
- Provide a method to modify the field values
- Getter and Setter method
- How to create an Immutable class?
All the fields should be made private so that direct access is not allowed
No setter methods
Make all mutable fields final, so that they can be assigned only once.
- How do you create a singleton class in java?
- A singleton class is a class that can have only one object (an instance of the class) at a time.
- After the first time, if we try to instantiate the Singleton class, the new variable also points to the first instance created.
- Make constructor as private.
- Write a static method that has return type object of this singleton class.
- Map Vs Flat Map?
Stream Vs parllelStreamList<List<String>> lists = Arrays.asList(Arrays.asList("1","2"),Arrays.asList("3"),Arrays.asList("33","44"));
System.out.println("FlatMap"+lists.stream().flatMap(lists2 -> lists2.stream()).collect(Collectors.toList()));
lists.stream().map(lists2 -> lists2.stream().collect(Collectors.toList())).forEach(System.out::println);
It is a way of logically grouping classes that are only used in one place: If a class is useful to only one other class, then it is logical to embed it in that class and keep the two together . Nesting such "helper classes" makes their package more streamlined.
Use a non-static nested class (or inner class) if you require access to an enclosing instance's non-public fields and methods. Use a static nested class if you don't require this access.
- Inner classes are used to get functionality that can get an object better than the method.
- They can be used in the case when a set of multiple operations are required and chances of reusability are good inside the class and they will not be accessed but methods outside The Outer Class.
- Inner Classes Are Made To Achieve Multiple Inheritances Also.
- Inner Classes Are Used When They Are Useful In The Class Context.
- They Are Used To Separate Logic Inside Classes.
- Inner Class Means One Class That Is A Member Of Another Class. There are basically four types of inner classes in java.
1) Nested Inner class
2) Method Local inner classes
3) Anonymous inner classes
4) Static nested classes
- Polymorphism
- Same name with different form dynamic and static polymorphism
- Static Polymorphism: Overload
- Dynamic polymorphism: Override
- Refer this link for more information:
- https://krishnababug.blogspot.com//2013/12/polymorphism-java-examples-static.html
- Overloading Vs Overriding
These two methods would have the same signature, but different implementation. One of these would. Method overriding means having a different implementation of the same method in the inherited class. These cannot exist in the same class.
Overriding methods
Overriding method definitionsIn a derived class, if you include a method definition that has the same name and exactly the same number and types of parameters as a method already defined in the base class, this new definition replaces the old definition of the method.
Explanation
A subclass inherits methods from a superclass. Sometimes, it is necessary for the subclass to modify the methods defined in the superclass. This is referred to as method overriding. The following example demonstrates method overriding.
- Constructor
- It will invoke when an object is instantiated.
- Will have same name as class name.
- This will not have any return type. Not even void.
- It will be called only once when an object is created.
- It cannot be override.
- It can be overload.
- Interface Vs Abstract class
- Final
- Members, Variables are final means Constant
- Method is final means cant override
- Class is final means cant inherit ie cant extend
- Finally
- The code which needs to executed before even if any exception occurs or not.
- Finalize
- If any resource needs to be freeze before the object gets destroyed we can use finalize Garbage collector internally call finalize.
- Inheritance
- Taking the properties of another class
- Encapsulation
- Wrapping the member and method with the use of access specifiers to prevent from the outside use
- Exceptions: T hrowable >>
- Error (checked exception) compile time exception no such Method, class not found exception
- Exception (unchecked exception) runtime exception Index out of bounce, Null pointer exception
- Try: Try:
- Nested try is allowed try can have either catch or finally
- Exception should be on top and IOException subclass should be in down that the order .
- try {} catch (IOException) {} catch (Exception)
- Catch: Catches the exception and handle it.
- Finally: It will execute the code no matter whether the exception is catches or not .
- Throw: To manually throw an exception
- Throws: Any exception that will throws by an system built-in exception .
- Threads : Running multiple process in a sequence .
- Threads shares same heap memory but different stack memory
- Two ways of creating threads: Extends thread and Implements Runnable Interface
- What are the different states of a thread's life-cycle? Ans) The different states of threads are as follows:
1) New – When a thread is instantiated it is in New state until the start () method is called on the thread instance. In this state the thread is not considered to be alive.
2) Runnable – The thread enters into this state after the start method is called in the thread instance. The thread may enter into the Runnable state from Running state. In this state the thread is considered to be alive.
3) Running - When The Thread Scheduler Picks Up The Thread From The Runnable Thread'S Pool, The Thread Starts Running And The Thread Is Said To Be In Running State.
4) Waiting / Blocked / Sleeping – In these states the thread is said to be alive but not runnable. The thread switches to this state because of reasons like wait method called or sleep method has been called on the running thread or thread might be waiting for some i / o resource so blocked.
5) Dead – When the thread finishes its execution ie the run () method execution completes, it is said to be in dead state. A dead state can not be started again. If a start ( ) method is invoked on a dead thread a run-time exception will occur. - Sleep Vs Wait?
1) wait is called from synchronized context only while sleep can be called without synchronized block. 2) wait is called on Object while sleep is called on Thread.
3) waiting thread can be awake by calling notify and notifyAll while sleeping thread can not be awaken by calling notify method.
4) wait is normally done on condition, Thread wait until a condition is true while sleep is just to put your thread on sleep.
5) wait release lock on object while waiting while sleep doesn' t release lock while waiting.
6) The notifyAll () method must be called from a synchronized context. - What is garbage collection? What is the process that is responsible for doing that in java?
Ans: Reclaiming the unused memory by the invalid objects. Garbage collector is responsible for this process. - What kind of thread is the Garbage collector thread?
Ans. It is a daemon thread. - What is a daemon thread?
Ans: These are the threads which can run without user intervention. The JVM can exit when there are daemon threads by killing them abruptly. - Synchronization: It is the process of making only one thread to access the resource at a time by using synchronized (this).
- we can use synchronize block also to avoid the deadlock condition
- String Vs StringBuffer: Read more about this here : http://www.krishnababug.com/2013/12/string-is-immutable-string-vs-string.html
- Static class loading: Class will be loaded statically with new operator
- Dynamic class loading: Class.forName (); string class name
- Connection pool:
- It's a technique to allow multiple clinets to make use of a cached set of shared and reusable connection objects providing access to a database.
- Opening / Closing database connections is an expensive process and hence connection pools improve the performance of execution of commands on a database for which we maintain connection objects in the pool.
- It facilitates reuse of the same connection object to serve a number of client requests.
- Every time a client request is received, the pool is searched for an available connection object and it's highly likely that it gets a free connection object.
- It's normally used in a web-based enterprise application where the application server handles the responsibilities of creating connection objects, adding them to the pool, assigning them to the incoming requests, taking the used connection objects back, returning them back to the pool, etc. ..
- Wrapperclass: As the name says, a wrapper class wraps (encloses) around a data type and gives it an object appearance. Wherever, the data type is required as an object, this object can be used. Wrapper classes include methods to unwrap the object And give back the data type. It can be compared with a chocolate. The manufacturer wraps the chocolate with some foil or paper to prevent from pollution. The user takes the chocolate, removes and throws the wrapper and eats it.
- Example: Example:
- int k = 100;
- Integer it1 = new Integer (k);
- The int data type k is converted into an object, it1 using Integer class. The it1 object can be used in Java programming wherever k is required an object.
- Session tracking:
- By using URL Rewriting
- Using session object
- Using cookies
- Using hidden fields
- Collections : Read this article for more information on collecitons http://www.krishnababug.com/2013/12/collections-java.html
- Sorting list in collection --using sort method: refere this article for more information: http://www.krishnababug.com/2014/01/sorting-list-using-comparator.html
- JSP Scripting elements:
- JSP scripting elements enable you insert Java code into the servlet that will be generated from the current JSP page.
- There are three forms:
- Expressions of the form <% = Java_expression%> that are evaluated and inserted into output, Example: Your hostname: <% = request.getRemoteHost ()%>
- Scriptlets of the form <% java_code%> that are inserted into the servlets service method, and Example: <%
String name = request.getParameter ("name");
out.println ("name:" + name);
%> - Declarations of the form <%! Java_code%> that are inserted into the body of the servlet class, outside of any existing methods. Example: <%!
public int getValue () {
return 78;
}
%> - Difference between JSP & Servlet: Servlet is html in java, JSP is java in html. JSP is a webpage scripting language that can generate dynamic content while Servlets are Java programs that are already compiled which also creates dynamic web content.In MVC, jsp act as a view and servlet act as a controller.
- What is the servlet lifecycle?
Ans:
Instantiation and initialization
The servlet engine creates an instance of the servlet. The servlet engine creates the servlet configuration object and uses it to pass the servlet initialization parameters to the init () method. The initialization parameters persist until The servlet is destroyed and are applied to all invocations of that servlet. Servicing requests
The servlet engine creates a request object and a response object. The servlet engine invokes the servlet service () method, passing the request and response objects.
The service () method gets information about the request from the request object, processes the request, and uses methods of the response object to create the client response. The service method can invoke other methods to process the request, such as doGet (), doPost (), or methods you write.
Termination After a servlet's destroy () method is invoked, the servlet engine unloads the servlet, and the Java virtual machine eventually performs garbage collection on the memory resources associated with the servlet.
The servlet engine stops a servlet by invoking the servlet's destroy () method. Typically, a servlet's destroy () method is invoked when the servlet engine is stopping a Web application which contains the servlet. The destroy () method runs only one time during the lifetime of the servlet and signals the end of the servlet. - Design patterns: Refer this link for more information on designpatterns: http://www.krishnababug.com/2014/01/design-pattern-interview-questions.html
- Webservices:
- Web services are Web based applications that use open, XML-based standards and transport protocols to exchange data with clients. Web services are developed using Java Technology APIs Web services are Web based applications that use open, XML-based standards and transport protocols to exchange data. with clients. Web services are developed using Java Technology APIs.
- Web services are client and server applications that communicate over the World Wide Web's (WWW) HyperText Transfer Protocol (HTTP). As described by the World Wide Web Consortium (W3C), web services provide a standard means of interoperating between software applications running on a web services are characterized by their great interoperability and extensibility, as well as their machine-processable descriptions, thanks to the use of XML.
- Simple Webservice code for online Webservice Stock Quote
- Hibernate:
- Hibernate is an object-relational mapping (ORM) library for the Java language, providing a framework for mapping an object-oriented domain model to a traditional relational database. Hibernate solves object-relational impedance mismatch problems by replacing direct persistence-related database accesses with high-level object handling functions.
- Hibernate's primary feature is mapping from Java classes to database tables (and from Java data types to SQL data types). Hibernate also provides data query and retrieval facilities. It generates SQL calls and relieves the developer from manual result set handling and object conversion. Applications using Hibernate are portable to supported SQL databases with little performance overhead
- If you are designing the database schema and your domain objects afresh.
- You envision that your domain objects and your schema will be in sync, for example an automobile vendor application where your vehicle objects are likely to be close to your table structures. Just an example.
- You really want to use an ORM and tie your objects closely to schema.
- Want to use a ORM framework to handle connections etc.
- Struts:
- Apache Struts is a web page development framework and an open source software that helps developers build web applications quickly and easily. Struts combines Java Servlets, Java Server Pages, custom tags, and message resources into a unified framework.
- Struts is based on famous MVC design pattern. The role played by Structs is controller in Model / View / Controller (MVC) style. The View is played by JSP and Model is played by JDBC or generic data source classes. set of programmable components that allow developers to define exactly how the application interacts with the user.
- core classes of Struts: Action, ActionForm, ActionServlet, ActionMapping, ActionForward are basic classes of Struts.
- configuration files used in Struts: ApplicationResources.properties, struts-config.xml .These two files are used to bridge the gap between the Controller and the Model.
- J DBC: Http://Www.Krishnababug.Com/2014/02/jdbc-java-interview-questions.Html
- SQL Injection : http://www.krishnababug.com/2014/02/sql-injection-java.html
- xcfd
Popular Posts
-
The best solution to know about these init levels is to understand the " man init " command output on Unix. There are basically 8...
-
How to Unlock BSNL 3G data card to use it with Airtel and Vodafone Model no : LW272 ? How to unlock BSNL 3G data card( Model no : LW272 )us...
-
How to transfer bike registration from one State to other (Karnataka to Andhra)?? Most of us having two wheelers purchased and registered in...
-
ఓం శ్రీ స్వామియే శరణం ఆయ్యప్ప!! Related posts : Trip to Sabarimala - Part 1 Trip to Sabarimala - Part 2 Ayappa Deeksha required things...
-
Following are some of interesting blogs I found till now ...please comment to add your blog here. Blogs in English : http://nitawriter.word...
Popular posts
- Airtel and vodafone GPRS settings for pocket PC phones
- Andhra 2 America
- Ayyappa Deeksha required things
- Blogs I watch !
- Captions for your bike
- DB2 FAQs
- Deepavali Vs The Goddes of sleep
- ETV - Dhee D2 D3
- Evolution of smoking in India Women
- How to make credit card payments?
- init 0, init 1, init 2 ..
- Java-J2EE interview preparation
- mCheck Application jar or jad download
- My SQL FAQs
- My Travelogues
- Old is blod - New is italic
- Online pay methids for credit cards
- Oracle FAQs
- Pilgrimages
- Smoking in Indian Women
- Technology Vs Humans
- Twitter feeds for all Telugu stars on single page.
- Unix best practices
- Unix FAQs